Enabling License Validation
We provide the flexibility to have different logical groupings of files for an add-on which we call "plans". Plans may be used for anything from organizing versions of your add-on to releasing different pricing bundles.
Licensing validation is a great way to ensure that your customers are always up-to-date on their payments. No having to chase them around to renew their purchase. We handle all of that automatically on your behalf. With it enabled it also gives you insight into some key information so that you will know exactly which host url, sugar version, sugar edition and more that they are using. This is both essential for providing top-notch (and even proactive) support and also to see the type of customers that are using your products.
If your plan"s pricing is subscription based (monthly/yearly), per-user based, or offers a free trial then using the License API is required. You can turn on the license validation for any plan by editing the add-on plan and setting "Require a License Key" to yes. Upon save you will see your add-on plan"s key. This is the key that you would then include in your distribution. See below for more information on how to include the key and how to add validation hooks to your add-on.
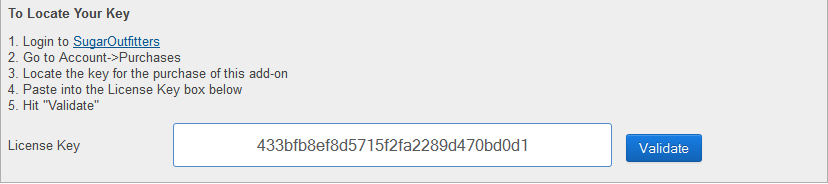
User Count Validation
If your add-on billing structure is "Per User" you can optionally turn on "Require User Count Verification" for the add-on plan. Without it, the purchaser will only be prompted for the user count at the time of the purchase. With it, you can add validation from your add-on within SugarCRM to see if the purchaser is under the user count that they have paid for. In addition, you can make it easy for the purchaser to add additional users to their purchase right from within their install. For example, if you are doing a marketing-like add-on you may wish to only count a subset of users who will actually be using it.
License for Specific Users
Many times you will only want to charge for those who use your add-on. We provide a user management tool so that a SugarCRM admin can specify which users can use your add-on and purchase additional licenses when more are needed. All right from within SugarCRM.
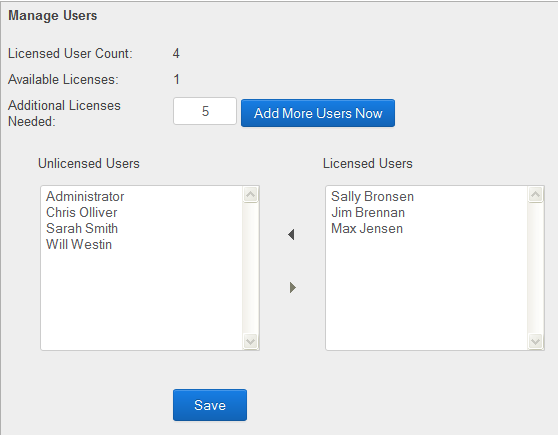
Adding License Validation to Your Add-on
Current Version: 2.1
Last Updated: 2024/11/13
Grab SampleLicenseAddon-cURL.zip if you are supporting cURL or SampleLicenseAddon-ExternalResourceClient.zip for Sugar's ExternalResourceClient.. This sample module includes a library that talks back to SugarCRM Marketplace, an admin configuration page for you module used to validate a license, and a post-install redirect to the config page to make configuring a module easy after installation. Alter it however you would like. For example, you could have ask the user for more information at the time of entering the license key or change the sample code to support dual licensing.
This Sample License Add-on can be installed as-is right into a SugarCRM install. Try it out by altering the shortname and public_key as described below for your add-on and see how it works. Post install you will be redirected right to the license configuration (and user management tool, if enabled).
To configure for your add-on edit /license/license/config.php (Note: /license/license gets copied into the module"s directory as just /license):
$outfitters_config = array(
"name" => "SampleLicenseAddon",
"shortname" => "samplelicenseaddon",
"public_key" => "",
"api_url" => "https://marketplace.sugarcrm.com/api/v1",
"validate_users" => false,
"manage_licensed_users" => false,
"validation_frequency" => "weekly",
"continue_url" => "",
);
- name The name of the Add-on should be the same as the name of your add-on in your manifest.php file. Adding this will allow you to see exactly which version of your add-on your customer is using when they validate their license.
- shortname The short name of the Add-on. e.g. For the url https://marketplace.sugarcrm.com/addons/sugaroutfitters the shortname would be sugaroutfitters
- public_key The public key associated with the plan. If you have multiple plans that all share the same code then you can pass a comma separated list of the public keys from each plan.
- api_url The Marketplace API url
- validate_users true if your add-on plan requires user count validation
- manage_licensed_users true to enable the user management tool to determine which users will be allowed to use the add-on
- validation_frequency How often should the license validate against the Marketplace License API? Options are hourly, daily, and weekly [default]
- continue_url [optional] Will show a button after license validation that will redirect to this page. Could be used to redirect to a configuration page such as index.php?module=MyCustomModule&action=config
Skipping public_key validation
There are situations where you'd like to maintain one single package to manage your licenses and have other packages with your functional code. For example, you have 8 different addon packages, each containing different logic and will be sold in different listings as well as have different public_keys for validation. In the conventional method, you'd have to replicate all the SampleLicenseAddon logic for each package, however, with this approach, you can create a 9th package that contains the logic to validate your functional packages. Here's how you'd package and distribute them:
- my-license-validation-addon.zip - contains validation logic that interacts with Marketplace (no public key at all)
- functional-addon-1.zip - contains your addon logic that the customer is interested in (contains valid public_key)
- functional-addon-2.zip - contains your addon logic that the customer is interested in (contains valid public_key)
- functional-addon-3zip - contains your addon logic that the customer is interested in (contains valid public_key)
When your customer buys your addon, they'd need to download 2 packages, one with the validation logic and the addon itself.
Your license validation is seen as a helper package and should not contain any public_key
as it does not work on its own.
SugarCRM Marketplace has a new capability that helps you to skip validation for this particular package at your disposal.
You must create a new PHP file, preferably under scripts folder with the following instructions:
cat scripts/ignore_license.php
<?php #SKIP-MARKETPLACE-PUBLIC-KEY-VALIDATION
When you upload your package, we will look for this Magic Key and if found, we will ignore public_key
validation and allow the package to be uploaded as a helper package for your functional packages. SugarCRM Marketplace still validates public_key
for other packages if that key is not found in the package.
Where and How Often Should You Validate?
Even if you are charging just a one-time, flat-rate fee you should be validating an installation"s license key on an on-going basis.
The SampleLicenseAddon.zip comes with helpers that will make it easy to check to see if a key is still active or not. You just need to find the right touch points in your code where it makes sense to trigger a license key check. If it fails, you then can proceed to notify the user and alter functionality as you feel is appropriate.
For example, if your add-on includes a standalone module you may wish to validate the key at the point of someone accessing that module. Or if your add-on is a Twitter dashlet you may validate the key whenever the dashlet is loaded. Your check may then look like this:
require_once("modules/SampleLicenseAddon/license/OutfittersLicense.php");
$validate_license = MarketplaceLicense::isValid("SampleLicenseAddon");
if($validate_license !== true) {
if(is_admin($current_user)) {
SugarApplication::appendErrorMessage("SampleLicenseAddon is no longer active due to the following reason: ".$validate_license." Users will have limited to no access until the issue has been addressed.");
}
echo "< h2>< p class="error">SampleLicenseAddon is no longer active< p class="error">Please renew your subscription or check your license configuration.";
//functionality may be altered here in response to the key failing to validate
}
Then you may choose to disable the feature until the key is entered in the License Configuration tool. MarketplaceLicense::isValid() in the SampleLicenseAddon that is provided will check the last validation result that is cached. If enough time has passed, per your validation_frequency setting, it will then connect to the License API to refresh the cached value. However, whenever anyone uses the License Configuration screen to update the key that always connects to the License API to get the latest result.
How to Validate for a Specific User
If you are using validate_users and manage_licensed_users then you can validate for a specific user by passing the user_id as the second parameter to the isValid check:
global $current_user;
require_once("modules/SampleLicenseAddon/license/MarketplaceLicense.php");
$validate_license = MarketplaceLicense::isValid("SampleLicenseAddon",$current_user->id);
if($validate_license !== true) {
echo "< h2>< p class="error">SampleLicenseAddon is no longer active< p class="error">".$validate_license."";
//functionality may be altered here in response to the key failing to validate
}
How to Test (via Add-on)
Under each pricing plan there is a Public Key (what you put into your license config) and a Customer Key. The Customer Key is what you will use to test your add-on as if you are the customer. Copy and paste this key into the license validation after your add-on is installed. You can see the latest successful ping under your Demo Keys page. From this page, you can suspend and unsuspend the key to test handling if the Customer Key does not successfully validate, mimicking an invalid or expired trial or subscription.
- Copy your Customer Key from the pricing plan
- Install your add-on
- Validate the license with your Customer Key
- View results on your demo keys page
Important note: Successful pings are stored in the config table in the CRM. Use the following SQL command to remove all cached license pings in order to get a fresh license validation test:
delete from config where category = "Marketplace" and name not like "lic%";
How to Test (Directly)
If you want a more direct way to test whether a license is valid other than by just using your add-on with licensing enabled you can hit a specific URL to see if the license is currently valid and also if a given user is also actively licensed (if using manage_licensed_users).
Test URL - Sugar 7 and up
#bwc/index.php?module=SampleLicenseAddon&action=marketplacecontroller&method=test&to_pdf=1
Test URL - Sugar 6
index.php?module=SampleLicenseAddon&action=marketplacecontroller&method=test&to_pdf=1
Want to test for a specific user? Simply add a user_id param:
#bwc/index.php?module=SampleLicenseAddon&action=marketplacecontroller&method=test&to_pdf=1&user_id=7eef99dd-5b60-1a5b-c123-53ac8394d21b
Avoiding Conflicts
To help ensure that MarketplaceLicense isn"t going to conflict with some other add-on"s version it is required to rename the class to the name of your module. For example, SampleAddonMarketplaceLicense. Your license validation would then use SampleAddonMarketplaceLicense::isValid("SampleLicenseAddon");
This will be deprecated and unneeded in the future when the Marketplace in-app management/store add-on is released.
Conclusion
With the SampleLicenseAddon example it should be fairly quick and easy to get up and running. As for how each add-on handles license validation (on each page request, upon login, once a week, etc along with what to do if validation fails) we leave that up to each developer as everyone has their own preference. If you find something that works well for you let us know!